In our previous article, we have discussed the difference between value type and reference type in c#.In this article let us explore c# class.
C# Class
C# is an OOP Language. A class is having fields as well as methods or actions which use these fields. So we are grouping fields and actions in a single unit. We can limit the accessibility of the fields and methods using access modifiers. In the real-world, to represent an entity we use classes. For example, we need to have student details in a school. A student will be having a Name, roll number, Department, etc. So we can create a class with the name “Student”.
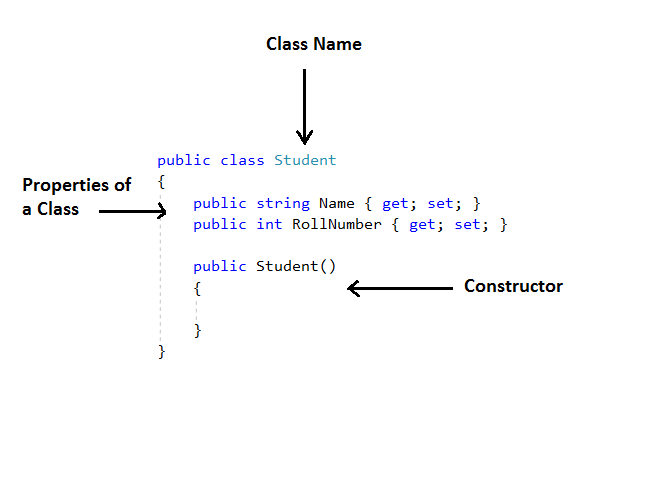
Access Modifiers
We can control the visibility of the property of a class. We can limit the accessibility of the property of the class to only inside the class or outside the class or for the derived class. When we make a property Public. It is accessible everywhere. A derived class also can read or modify these properties. When we mark property with Private the property is visible only inside the containing class, which means we cannot access this property outside the class. You might think why would anybody need a Private access modifier, It is to protect the data from modifications from the entity from outside of this class. Imagine a Banking application where we want to secure Balance Details, Card details, and other transaction details. We have Protected access modifiers its protection level stands in between private and public. A variable with a protected access modifier is available inside the same class just like private property and inside a class that is derived from it. Similarly, we can limit the accessibility of the method inside the class using private and public access modifiers. Below is an example of a private and public variable in a class.
public class Employee { public string Name; private int _salary { get; } }
Constructor
Constructor in a class is helpful to initialize the class variables on creating an object of that class. A constructor is just like a method of a class but the name of the method should be the same as class. We can have more than one constructor for class with varying signature. If we don’t provide a constructor explicitly to a class, then c# provided a default constructor.
Constructors are not only limited to initializing the values but useful to perform operations such as dependency injection. Constructors should be having access modifiers as public otherwise we cannot create the object of a class. The below example explains how to create a constructor in a class.
using System; namespace MyTestConsoleApp { class Practice { public class Employee { public string Name; private int _salary { get; } //Constructor 1 public Employee(int salary) { this._salary = salary; } //Constructor 2 public Employee(string Name, int salary) { this.Name = Name; this._salary = salary; } } public static void Main(String[] args) { Employee emp1 = new Employee(500); emp1.Name = "Hari"; Employee emp2 = new Employee("Hari", 500); Console.ReadLine(); } } }
Static parameters in C# Class
Static parameters in a class can be accessed using the class name. The static parameter is shared across all the instances of a class, which means if we have 10 objects of a class which all will point to the same static variable in memory. Static variables can be initialized using a Static constructor which will be executed only once and it will be during the first instantiation of the object. Static parameters can be used when we have a variable that will be common for all. Below is a simple class circle with radius and pi
as parameters. Here pi
is declared as a static parameter and initializing the value in the static constructor.
using System; namespace Learn { public class Circle { public static float pi; private float _radius; public Circle(float radius) { this._radius = radius; } static Circle() { pi = 3.14f; } public float Area() { return pi * _radius * _radius; } } class Learn { public static void Main(String[] args) { Circle c = new Circle(5); Console.WriteLine(c.Area()); //78.5 } } }
Getters and Setters in C# Class
Properties in C# are used to read, write to private fields securely. We can expose the private field of a class to outside via properties. Using “Set” property allows a private field to behave just like a public variable when we assign values. Similarly, we can control the accessibility of a variable using “get” property. So with the help of this, we can limit the variable read-only, write-only, and read & write. Below is an example of how we can use C# property to have better control over the private variables.
using System; namespace MyTestConsoleApp { class Practice { public class Student { private int _totalMarks; private int _rollNumber; private int _percentage; private int _age; public string Name { get; set; } public Student(int rollNumber,int totalMarks) { this._rollNumber = rollNumber; this._totalMarks = totalMarks; this._percentage= (_totalMarks * 100) / 1000; ; } //readonly public int Percentage { get { return this._percentage; } } //read and customizd write public int Age { set { if(value < 100) { this._age = value; } else { throw new Exception("Age should be below 100"); } } get { return this._age; } } } public static void Main(String[] args) { Student student = new Student(1234, 560); student.Name = "Ram"; student.Age = 25; Console.WriteLine(string.Format("Name={0},Age={1},Percentage={2}", student.Name, student.Age, student.Percentage)); Console.ReadLine(); } } }