In C# there are two types of variables one is Value Type and the other one is a Reference type. In this article, we will see these two in detail.
Value Type
In C# variable of value type contains the value of the type.int, float, char, etc are examples of this. In this type when you copy the value from one variable to another variable only the value copied and they are two independent variables.
Example:
int x=10;
int y=20;
x=y;
public static void Main(String[] args) { int x = 10; int y = 20; int? z = 100; x = y; //value is copied x = (int)z; //explicty converted to non nullable from nullable. }
We can not assign null to a variable of this type. But in some cases, we have to store null values in these variables. We can make this nullable by using the “?” operator.int? x=null;
when we assign a nullable type to a non-nullable, we need to explicitly do it.
Example:
int? x=null;
int y=20;
y=(int)x;
A struct is a value type, In the below example, Rectangle is a struct with length and breadth properties. It also has a method called Area which returns the Area of the rectangle. After assigning r1 to r2, the value is copied and both are independent instances, modifying the properties of r1 did not change the same in r1.
using System; namespace Learn { class Learn { public struct Rectangle { public int breadth; public int length; public Rectangle(int b, int l) { this.breadth = b; this.length = l; } public long Area() { return breadth * length; } public override string ToString() { return $"Length={length},Breadth={breadth},Area={Area()}"; } } public static void Main(String []args) { Rectangle r1 = new Rectangle(10,20); Rectangle r2 = r1; r2.breadth = 15; Console.WriteLine(r1); Console.WriteLine(r2); } } }
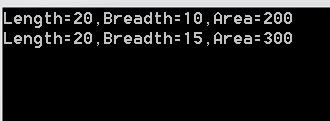
Reference Type
The reference type holds the reference to an instance object not the object. So when we copy the one reference variable to another the reference is copied. string,object etc are reference types. Instances of class,interface,delegate are of Reference type.
public static void Main(String[] args) { //Reference Types Student s1 = new Student(); s1.Name = "Hari"; s1.RollNumber = 1234; Student s2 = new Student(); s2 = s1; //reference is copied here s2.Name = "krishna";//so changes made to s2 will reflect in s1 also. Console.ReadLine(); }
Ref Keyword
We can also pass a value type by reference using ref keyword. In that case whatever changes that we make for that variable inside the called method, which will also be reflected in the calling method.
In the below example we are passing variable x
to the function Double
by reference. Inside the function, we multiply it by 2, and the change also reflects in the main method.
using System; namespace Learn { class Learn { public static void Double(ref int x) { x = 2 * x; } public static void Main(String[] args) { int x = 10; Double(ref x); Console.WriteLine(x);//20 } } }
Ref Keyword with Reference Type
The below code explains how we can pass by reference for a Reference type. We have overloaded a method AddStep
, one with a pass by reference & the other is direct. In pass by reference, we are assigning a new instance of the class Plane
with different values for x
and y
, after the call to AddStep
the p in the main method or calling method also points to a new location.
using System; namespace Learn { public class Plane { public int x; public int y; public Plane(int X,int Y) { this.x = X; this.y = Y; } public override string ToString() { return $"x={x},y={y}"; } } class Learn { public static void AddStep(Plane p) { p = new Plane(20, 30); } public static void AddStep(ref Plane p) { p = new Plane(20, 30); } public static void Main(String[] args) { Plane p = new Plane(10, 20); Console.WriteLine(p);//x=10,y=20 AddStep(p); Console.WriteLine(p);//x=10,y=20 Console.WriteLine("Pass by Reference"); AddStep(ref p); Console.WriteLine(p);//x=20,y=30 } } }
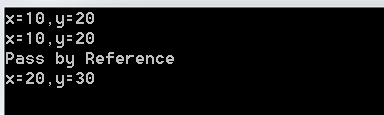
One thought on “C# Value Type and Reference Type”