Abstract Class in C# is similar to Interface but with some differences. In this article let us explore the features of abstract modifiers in detail with real-time examples.
Abstract Modifier in C#
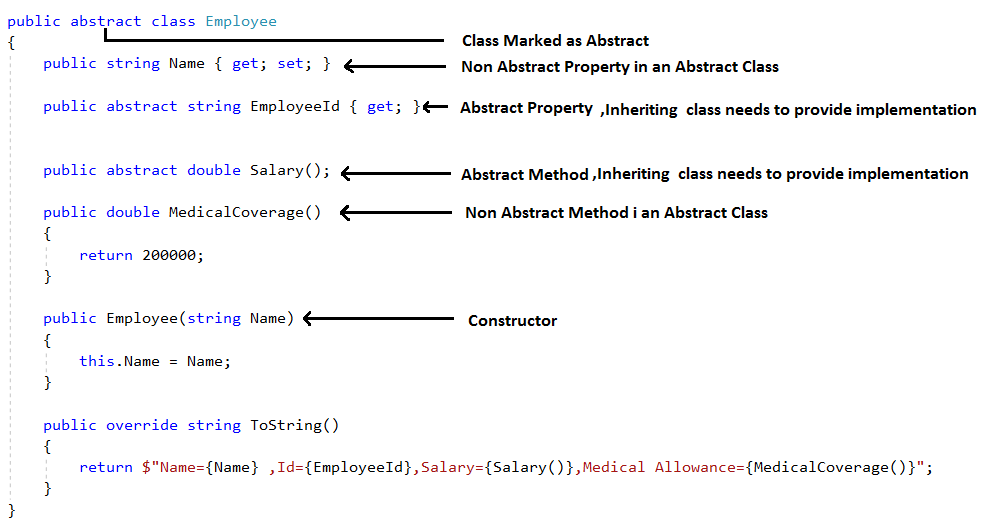
An abstract modifier is used in C# to tell that the information is incomplete or currently not available. Abstract keyword is used with classes, methods, properties, events, and indexers. When used with the class we call that class an abstract class. An abstract class is an incomplete class, which this class can’t be instantiated and a derived class should provide an implementation for its abstract members.
Abstract classes cannot be marked as a sealed class because abstract and sealed functionalities are contradicting each other. An abstract class can have an implementation for some of its members. It is the responsibility of the derived class to provide implementation to an abstract member, if it cant provide all implementation, then that class also should be marked as an abstract. A static & Virtual keyword cannot be used with a method that is marked as abstract. By using abstract classes, it is very easy to place the common functionality under one class, and all the other classes which share the common information can be made to inherit from this class. In design patterns, we will see abstract classes are frequently used to write reusable and maintainable code.
Abstract Class in C# with Example
The below example explains this in detail. Consider a real-world example. We could have Permanent & Contract employees in an organization, but both share common information such as Employee Name & Employee Id, etc. Instead of repeating this information, we can create an abstract class with the name “Employee” with a common property Name and Id. The employee has a salary also, but the calculation of salary may vary based on the type of employee. So, we can let the Permanent & Contract employee classes provide the custom logic for the same.
Here EmployeeId
property and Salary()
method is marked as abstract property and abstract method respectively. The implementing class needs to provide the implementation for these members. In our example below Permanent Employee Paid monthly whereas Contract Employee paid on an hourly basis. Also, the employee id of the contract employee starts with “TMP”.
using System; using System.Collections.Generic; namespace Learn { public abstract class Employee { public string Name { get; set; } public abstract string EmployeeId { get; } public abstract double Salary(); public double MedicalCoverage() { return 200000; } public Employee(string Name) { this.Name = Name; } public override string ToString() { return $"Name={Name} ,Id={EmployeeId},Salary={Salary()},Medical Allowance={MedicalCoverage()}"; } } public class PermanentEmployee : Employee { private string Id; public double MonthlySalary { get; } public override string EmployeeId { get { return "TMP" + Id; } } //override to provide Monthly Salary public override double Salary() { return MonthlySalary; } public PermanentEmployee(string Id,string Name,double MonthlySalary) : base(Name) { this.MonthlySalary = MonthlySalary; this.Id = Id; } public override string ToString() { return base.ToString(); } } public class ContractEmployee : Employee { private string Id; public double HourlyPay { get; } public override string EmployeeId { get { return Id; } } public override double Salary() { return HourlyPay; } public ContractEmployee(string Id, string Name,double HourlyPay) : base(Name) { this.HourlyPay = HourlyPay; this.Id = Id; } public override string ToString() { return base.ToString(); } } class Learn { public static void Main(string[] args) { Employee emp1 = new PermanentEmployee("267543", "Tom", 5000); Employee emp2 = new ContractEmployee("953456", "Ketty", 30); Console.WriteLine(emp1); Console.WriteLine(emp2); } } }
Let’s see a few key points summarized about Abstract class in C# from the above sections.
- It is not possible to create an instance of an abstract class.
- It has abstract properties, abstract methods, constructors, etc. but not static abstract members.
- An abstract class can have non-abstract members with implementation.
- A class inheriting an abstract class needs to provide an implementation for abstract members otherwise those members and class should be marked abstract.
- We cannot make an abstract class sealed.
Abstract Class and Interface in C#
As we already discussed abstract class in our previous sections, you may have a question in your mind about how it is different from Interface. Because some of the features look similar.
Let us see some of the key differences between Abstract class and Interfaces in C#.
- Abstract members can have access modifiers but the interface cannot have access modifiers.
- An abstract class can have a constructor but the interface cannot have a constructor.
- Class Implementing an Interface must provide an implementation for all its members. But a class inheriting an abstract class can be marked as abstract if it is not providing an implementation for abstract members.
- An abstract class can have implementations for some of its nonabstract properties or methods. But Interface cannot have an implementation (however new versions of C# supports default implementation).