What is an Exception?
Exceptions occur in a program when something disrupts the normal flow of the program. It could be due to an error inside the code written or when the specific condition met which is not handled properly. All developers would have encountered some error at least once when they run the new code or when they test the new code.
NullReferenceException is an example of an exception. It occurs when we try to invoke any operation on an object whose value is null.
When such an exceptional event or exceptions occurred it gives an object called exception object which has the details such as type, error message, the method from where the error occurred, information about the current state of the program, etc.
Exception in C#
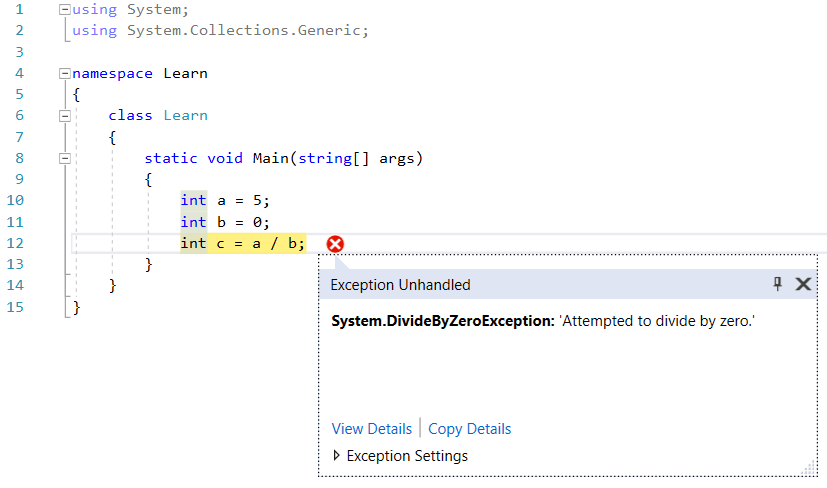
The above figure shows DivideByZeroException occurred when we tried to divide a number by zero. Since we did not handle the exception Visual Studio shows the unhandled exception & stops execution of the program.
The Exception Object is very helpful to deal with unforeseen situations where we may not have a proper logic to control the flow of a program or to just log such events. C# provides exception handling using try & catch block. An exception can be anything, say for example when we try to divide by zeros since we cannot do such operation computers don’t know what to do, so throws an exception “DivideByZeroException“.
Try & Catch in C#
Try block followed by Catch block used in C# to handle the exceptions.When a block of code expected to throw an exception due to some reason, we can wrap the code in a try block .Let us see an example of this by modifying previous code.
using System; using System.Collections.Generic; namespace Learn { class Learn { static void Main(string[] args) { try { int a = 5; int b = 0; int c = a / b; Console.WriteLine($"Result={c}"); } catch (DivideByZeroException) { Console.WriteLine("Cannot Divide a Number by Zero"); } } } }

In the above example, we are doing a division operation. We have wrapped the code in a try block. In the catch block, we are checking for the exception of type DivideByZeroException.We write the code to handle these errors in the catch block. In this example, we are writing a useful message to the console thereby letting the user know about this problem. Similarly, we can handle other exceptional scenarios in the catch block.
The order of the exception matters, we need to have all the specific exception at beginning of the catch block, exceptions of type Exception should be kept last which will be always caught in case of any exception
Finally Block in Try-Catch
In addition, to try and catch block we also have another block after catch block which is finally
block. This is an optional block this block will be executed always.
That means if the code execution results in an exception then after catch block finally block will be executed or if the execution succeeds then also finally block will be executed. Finally block usually has cleanup code such as closing a DB Connection, closing a file stream, etc.
Below is the example for try catch finally block. In this example, we are trying to read file content. We are using StreamReader
for reading a file. We are closing the stream object and underlying stream.
using System; using System.Collections.Generic; using System.IO; namespace Learn { class Learn { static void Main(string[] args) { StreamReader rdr = null; string result = string.Empty; try { rdr = new StreamReader("C:/Test/test.txt"); result = rdr.ReadToEnd(); Console.WriteLine("File Content=" + result); } catch (FileNotFoundException) { Console.WriteLine("File Specified is not found"); } catch (DirectoryNotFoundException) { Console.WriteLine("Directory Specified is not found"); } finally { if (rdr != null) { rdr.Close(); } } } } }
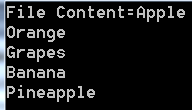
User Defined Exception in C#
DotNet Framework provides many built-in exceptions that we can use right away. All exceptions inherited from a class called “Exception”. Suppose if we want to create our own exception we can do so by creating a class that derives from the Exception class. By convention, the exception class names end with “Exception”. Below is the example for the custom exception in C#.
public class MyCustomException : Exception { public MyCustomException() { } public MyCustomException(string message) : base(message) { } public MyCustomException(string message, Exception inner) : base(message, inner) { } }
Throw New Exception in C#
We can use throw statement to throw new exceptions or to pass the exception to the caller method. Below code, line throws the exception of type MyCustomException.
throw new MyCustomException("custom exception message");
Let us see an example to see this in action.
using System; using System.Collections.Generic; using System.IO; namespace Learn { public class MyCustomException : Exception { public MyCustomException() { } public MyCustomException(string message) : base(message) { } public MyCustomException(string message, Exception inner) : base(message, inner) { } } class Learn { public static void DoSomeWork() { //other code throw new MyCustomException("custom exception message"); } static void Main(string[] args) { try { DoSomeWork(); } catch (MyCustomException ex) { Console.WriteLine($"Message={ex.Message}"); } } } }
