Partial Keyword in C# can be applied to Class, Interfaces, and Struct. By using the Partial keyword in a class, we can divide the class definitions and other properties into different files. When we compile the code, the class will combine all the partial code definitions related to the class into one class and compile. The different parts of the code must be under one namespace and assembly.
Use of Partial Class in C#
- By using this feature we can write code related to the same class at different places in code base when the multiple developers are working on different features.
- This is also helpful when IDE generates auto-generated code stubs, so it makes developers work easily.
- Maintaining Auto Generated code & User developed code is easy.
Important Points to Remember,
- Different parts of the code implementing a class/interface/struct should be marked as Partial.
- If one part of the partial code made to inherit a class or interface, the whole class inherits those classes or interfaces.
- If a partial class made as the abstract whole class becomes abstract, similarly to a sealed class.
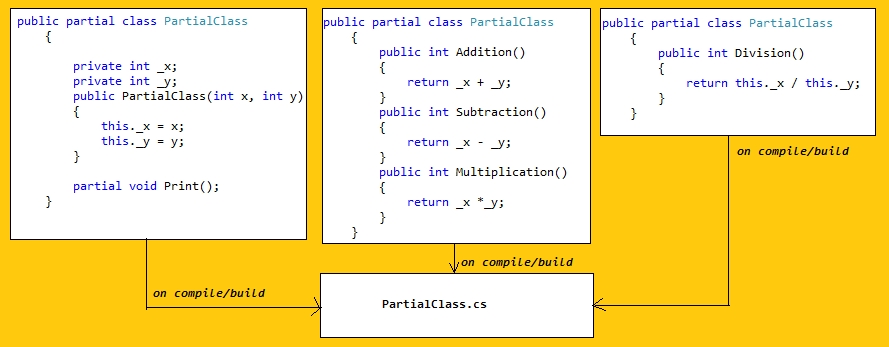
Let’s see a partial class in c# with example. We need to create a class, let’s call it PartialClass
, which does basic math operations such as addition, subtraction, etc. Imagine one developer working on class parameters & constructor, and others working on the math operation. The class spitted its definition across 3 files namely PartialClass1
,PartialClass2
& PartialClass3
. PartialClass1
contains private properties and constructors. PartialClass2
contains 3 math operations & finally, PartialClass3
contains one operation. When we compile this class compiler looks for all the related definition of the class and combines it to build. Let’s see the code,
PartialClass1.cs
nnamespace Learn { public partial class Maths { private int _x; private int _y; public Maths(int x, int y) { this._x = x; this._y = y; } } }
PartialClass2.cs
namespace Learn { public partial class Maths { public int Addition() { return _x + _y; } public int Subtraction() { return _x - _y; } public int Multiplication() { return _x * _y; } } }
PartialClass3.cs
public partial class Maths { public int Division() { return this._x / this._y; } } class Learn { public static void Main(string[] args) { Maths maths = new Maths(40, 20); Console.WriteLine($"Addition={maths.Addition()}"); Console.WriteLine($"Substraction={maths.Subtraction()}"); Console.WriteLine($"Multiplication={maths.Multiplication()}"); Console.WriteLine($"Division={maths.Division()}"); } }
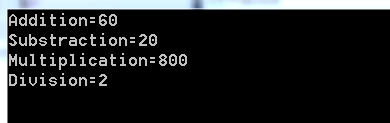
Partial Interface in C#
Let’s see another example for the partial Interface. Consider interface for logging messages on to the console. We can log the success message, error message. Based on this we can have 3 different methods.
We will have two partial interfaces one in File1.cs and another one in File2.cs
namespace Learn { public partial interface ILog { void LogSuccess(string message); } }
Another part of it looks like below,
namespace Learn { public partial interface ILog { void LogError(string message); void LogWarning(string message); } }
Class Log implements these interface,and this class has to provide implementation to all the 3 interface methods as shown below.
using System; using System.Collections.Generic; namespace Learn { public class Log : ILog { public void LogError(string message) { Console.WriteLine($"{DateTime.Now}|Error|{message}"); } public void LogSuccess(string message) { Console.WriteLine($"{DateTime.Now}|Success|{message}"); } public void LogWarning(string message) { Console.WriteLine($"{DateTime.Now}|Warning|{message}"); } } class Learn { public static void Main(string[] args) { ILog logger = new Log(); logger.LogSuccess("Success Message"); logger.LogError("Error Message"); logger.LogWarning("Warning Message"); } } }

Partial Method in C#
In C# method can also be partial. We can write the partial method signature in one file and the definition in another file. Developers can provide definitions or leave without providing the definition. In the latter case, the compiler won’t throw any error as the compiler removes that partial method & any references of it in the code. Below are some of the features of Partial Methods.
- The partial method is implicitly private.
- The return type of partial method is void.
- No Access modifiers allowed as the method is private.
- Our parameter is now allowed with the partial method.
- The partial method can be static as well.
Partial method if unimplemented won’t throw compiler error or runtime error. Let us verify this with an example. We will modify the previous example of partial class to show this.
partial void PartialMethodCall();
The above method declares a partial method and we are skipping the definitions for this function. But the below code runs without any problem.
using System; using System.Collections.Generic; namespace Learn { public partial class Maths { public int Division() { PartialMethodCall(); return this._x / this._y; } partial void PartialMethodCall(); } class Learn { public static void Main(string[] args) { Maths maths = new Maths(40, 20); Console.WriteLine($"Division={maths.Division()}"); } } }
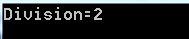